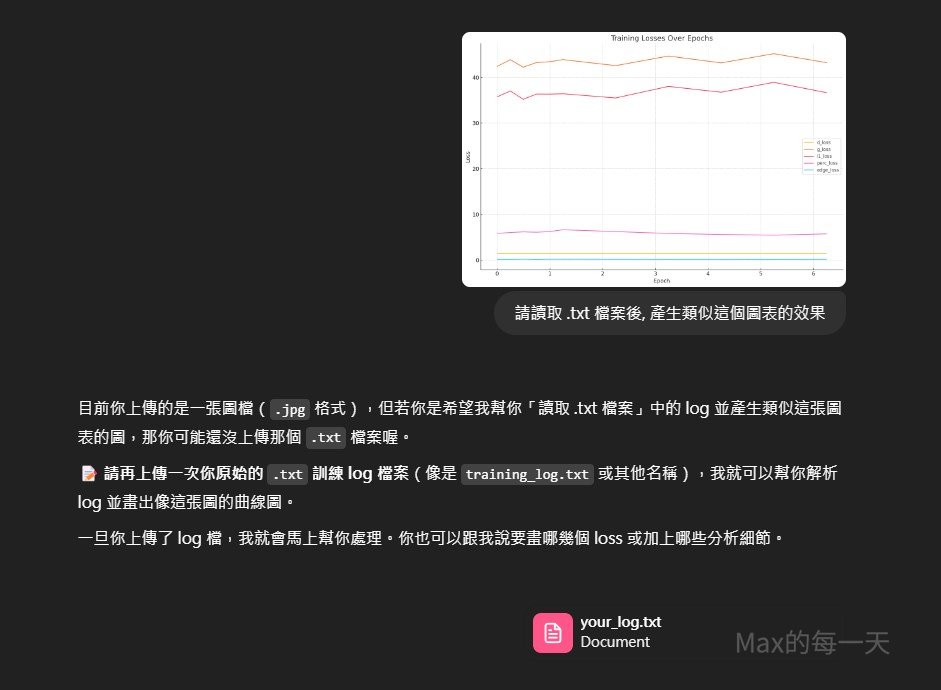
想將你的數據轉化為清晰易懂的視覺化圖表嗎?現在,這個過程變得前所未有的簡單!
方法是這樣的:先將你的 log 檔上傳給 ChatGPT,請它根據內容產製圖表。或者,也可以在網路上找尋其他人的成果範例圖片,把圖片上傳給 ChatGPT並下達這樣的提示詞:
請讀取 .txt 檔案後, 產生類似這個圖表的 python script
當然可以!以下是調整後的 plot_losses.py
,加入了命令列參數功能,讓你可以用如下方式執行並指定檔案名稱:
python plot_losses.py your_log.txt
✅ 修改後的 plot_losses.py
import re
import sys
import pandas as pd
import matplotlib.pyplot as plt
def parse_log_file(filepath):
with open(filepath, 'r') as file:
log_text = file.read()
pattern = re.compile(
r"Epoch: \[\s*(\d+)\], Batch: \[\s*\d+/\s*\d+\].*?\n"
r"\s*d_loss:\s*([\d.]+),\s*g_loss:\s*([\d.]+),\s*const_loss:\s*([\d.]+),\s*"
r"l1_loss:\s*([\d.]+),\s*fm_loss:\s*([\d.]+),\s*perc_loss:\s*([\d.]+),\s*edge:\s*([\d.]+)"
)
records = []
for match in pattern.finditer(log_text):
epoch = int(match.group(1))
d_loss = float(match.group(2))
g_loss = float(match.group(3))
const_loss = float(match.group(4))
l1_loss = float(match.group(5))
fm_loss = float(match.group(6))
perc_loss = float(match.group(7))
edge = float(match.group(8))
records.append({
'epoch': epoch,
'd_loss': d_loss,
'g_loss': g_loss,
'const_loss': const_loss,
'l1_loss': l1_loss,
'fm_loss': fm_loss,
'perc_loss': perc_loss,
'edge': edge
})
return pd.DataFrame(records)
def plot_losses(df):
df_mean = df.groupby('epoch').mean().reset_index()
plt.figure(figsize=(12, 7))
loss_types = ['d_loss', 'g_loss', 'l1_loss', 'perc_loss', 'edge']
for loss in loss_types:
plt.plot(df_mean['epoch'], df_mean[loss], label=loss)
plt.title('Training Loss Over Epochs')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.grid(True)
plt.tight_layout()
plt.show()
if __name__ == "__main__":
if len(sys.argv) < 2:
print("用法: python plot_losses.py <log檔案名稱>")
sys.exit(1)
log_file = sys.argv[1]
try:
df = parse_log_file(log_file)
plot_losses(df)
except FileNotFoundError:
print(f"找不到檔案: {log_file}")
except Exception as e:
print(f"發生錯誤: {e}")
🧪 使用方法:
python plot_losses.py your_log.txt
需要我幫你把結果存成 PNG 圖檔?或要加上滑動平均曲線?都可以幫你擴充 👍
更神奇的是,只需要一個「要」字,就能擴充功能!無論是將結果存成 PNG 檔,還是加上滑動平均曲線,ChatGPT 都能輕鬆搞定 👍
透過 ChatGPT 提供的程式碼產生的圖表,簡直令人難以置信!竟然無需任何程式基礎,就能獲得想要的圖表和對應的程式碼。從此以後,自己就能離線且隨時產生相同的圖表,再也不用麻煩 ChatGPT 來產生!
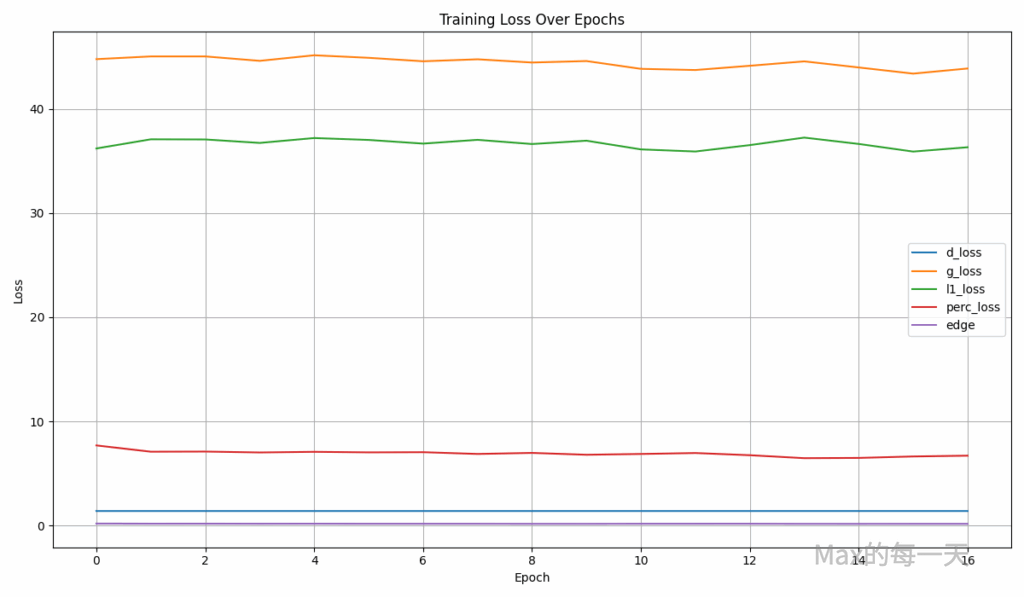
使用 AI 增強與調整功能:
好的,這是一個修改後的 script,使用 argparse
處理命令行參數,並增加了 -s/--silent
參數來控制是否顯示 plot,以及儲存圖檔的功能:
Python
import re
import argparse
import pandas as pd
import matplotlib.pyplot as plt
import os
def parse_log_file(filepath):
with open(filepath, 'r', encoding='utf-8') as file:
log_text = file.read()
pattern = re.compile(
r"Epoch: \[\s*(\d+)\], Batch: \[\s*\d+/\s*\d+\].*?\n"
r"\s*d_loss:\s*([\d.]+),\s*g_loss:\s*([\d.]+),\s*const_loss:\s*([\d.]+),\s*"
r"l1_loss:\s*([\d.]+),\s*fm_loss:\s*([\d.]+),\s*perc_loss:\s*([\d.]+),\s*edge:\s*([\d.]+)"
)
records = []
for match in pattern.finditer(log_text):
epoch = int(match.group(1))
d_loss = float(match.group(2))
g_loss = float(match.group(3))
const_loss = float(match.group(4))
l1_loss = float(match.group(5))
fm_loss = float(match.group(6))
perc_loss = float(match.group(7))
edge = float(match.group(8))
records.append({
'epoch': epoch,
'd_loss': d_loss,
'g_loss': g_loss,
'const_loss': const_loss,
'l1_loss': l1_loss,
'fm_loss': fm_loss,
'perc_loss': perc_loss,
'edge': edge
})
return pd.DataFrame(records)
def plot_losses(df, output_filename=None):
df_mean = df.groupby('epoch').mean().reset_index()
plt.figure(figsize=(12, 7))
loss_types = ['d_loss', 'g_loss', 'l1_loss', 'perc_loss', 'edge']
for loss in loss_types:
plt.plot(df_mean['epoch'], df_mean[loss], label=loss)
plt.title('Training Loss Over Epochs')
plt.xlabel('Epoch')
plt.ylabel('Loss')
plt.legend()
plt.grid(True)
plt.tight_layout()
if output_filename:
plt.savefig(output_filename)
print(f"圖檔已儲存至: {output_filename}")
else:
plt.show()
if __name__ == "__main__":
parser = argparse.ArgumentParser(description="從 log 檔案中解析 loss 並繪製圖表。")
parser.add_argument("log_file", help="輸入的 log 檔案名稱")
parser.add_argument("-s", "--silent", action="store_true", help="不顯示 plot,直接儲存圖檔")
args = parser.parse_args()
log_file = args.log_file
silent_mode = args.silent
try:
df = parse_log_file(log_file)
base_filename = os.path.splitext(log_file)[0]
output_filename = f"{base_filename}.png"
plot_losses(df, output_filename if silent_mode else None)
except FileNotFoundError:
print(f"找不到檔案: {log_file}")
except Exception as e:
print(f"發生錯誤: {e}")
主要修改說明:
- 引入
argparse
模組: Pythonimport argparse
- 設定 ArgumentParser: Python
parser = argparse.ArgumentParser(description="從 log 檔案中解析 loss 並繪製圖表。") parser.add_argument("log_file", help="輸入的 log 檔案名稱") parser.add_argument("-s", "--silent", action="store_true", help="不顯示 plot,直接儲存圖檔") args = parser.parse_args()
argparse.ArgumentParser()
創建一個解析器物件,用於定義和解析命令行參數。parser.add_argument("log_file", ...)
定義一個位置參數log_file
,使用者必須提供這個參數,它是 log 檔案的路徑。parser.add_argument("-s", "--silent", ...)
定義一個可選的 flag 參數-s
或--silent
。action="store_true"
表示當使用者提供這個 flag 時,args.silent
的值會是True
,否則為False
。
- 取得參數值: Python
log_file = args.log_file silent_mode = args.silent
從args
物件中取得log_file
和silent
的值。 - 修改
plot_losses
函數:- 增加一個可選參數
output_filename
。 - 如果
output_filename
有值,則使用plt.savefig(output_filename)
將圖表儲存到檔案,並印出儲存成功的訊息。 - 如果
output_filename
為None
(表示沒有提供,或者-s
參數未被使用),則呼叫plt.show()
顯示圖表。
- 增加一個可選參數
- 在
if __name__ == "__main__":
區塊中處理silent_mode
: Pythonbase_filename = os.path.splitext(log_file)[0] output_filename = f"{base_filename}.png" plot_losses(df, output_filename if silent_mode else None)
- 使用
os.path.splitext()
取得輸入 log 檔案的主檔名(不包含副檔名)。 - 根據主檔名建立
.png
檔案名稱。 - 將
output_filename
傳遞給plot_losses
函數。只有當silent_mode
為True
時,output_filename
才會有值,否則為None
。
- 使用
如何執行這個 script:
- 儲存檔案: 將修改後的程式碼儲存為一個
.py
檔案,例如plot_losses.py
。 - 在終端機中執行:
- 顯示 plot (預設行為): Bash
python plot_losses.py your_log_file.log
將your_log_file.log
替換為你的實際 log 檔案名稱。 - 不顯示 plot,直接儲存檔案: Bash
python plot_losses.py -s your_log_file.log
或者使用長參數: Bashpython plot_losses.py --silent your_log_file.log
這會在與 log 檔案相同的目錄下,產生一個名為your_log_file.png
的圖檔。
- 顯示 plot (預設行為): Bash
現在這個 script 更加靈活,可以透過命令行參數控制是否顯示 plot 並儲存圖檔。